Tags
ASP.NET Core provides several options for authentication, including cookie-based authentication, token-based authentication (using JWT), and external authentication providers such as Google, Facebook, and Twitter. Here's a brief overview of how to set up cookie-based authentication in ASP.NET Core:
Add authentication middleware to the application's
Startup.cs
file. This middleware can be added by calling theAddAuthentication()
method and specifying the authentication scheme you want to use (in this case, cookie-based authentication).public void ConfigureServices(IServiceCollection services)
{
// Add authentication services
services.AddAuthentication(CookieAuthenticationDefaults.AuthenticationScheme)
.AddCookie(options =>
{
options.LoginPath = "/Account/Login";
options.AccessDeniedPath = "/Account/AccessDenied";
});
}Configure the authentication middleware to use the cookie-based authentication scheme. This is done in the
Configure()
method ofStartup.cs
.public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// Add authentication middleware
app.UseAuthentication();
}Protect the desired routes or controllers with the
[Authorize]
attribute. This attribute can be placed at the controller or action level to restrict access to authorized users only.[Authorize]
public class HomeController : Controller
{
public IActionResult Index()
{
return View();
}
}Implement login and logout actions in your account controller. This is where the user's credentials are validated and a cookie is issued to the user upon successful authentication.
public class AccountController : Controller
{
[HttpGet]
public IActionResult Login()
{
return View();
}
[HttpPost]
public async Task<IActionResult> Login(LoginViewModel model)
{
// Validate user credentials and issue cookie
var result = await _signInManager.PasswordSignInAsync(model.Email, model.Password, model.RememberMe, lockoutOnFailure: false);
if (result.Succeeded)
{
return RedirectToAction("Index", "Home");
}
ModelState.AddModelError(string.Empty, "Invalid login attempt.");
return View(model);
}
[HttpPost]
public async Task<IActionResult> Logout()
{
// Sign out current user and remove authentication cookie
await _signInManager.SignOutAsync();
return RedirectToAction("Index", "Home");
}
}
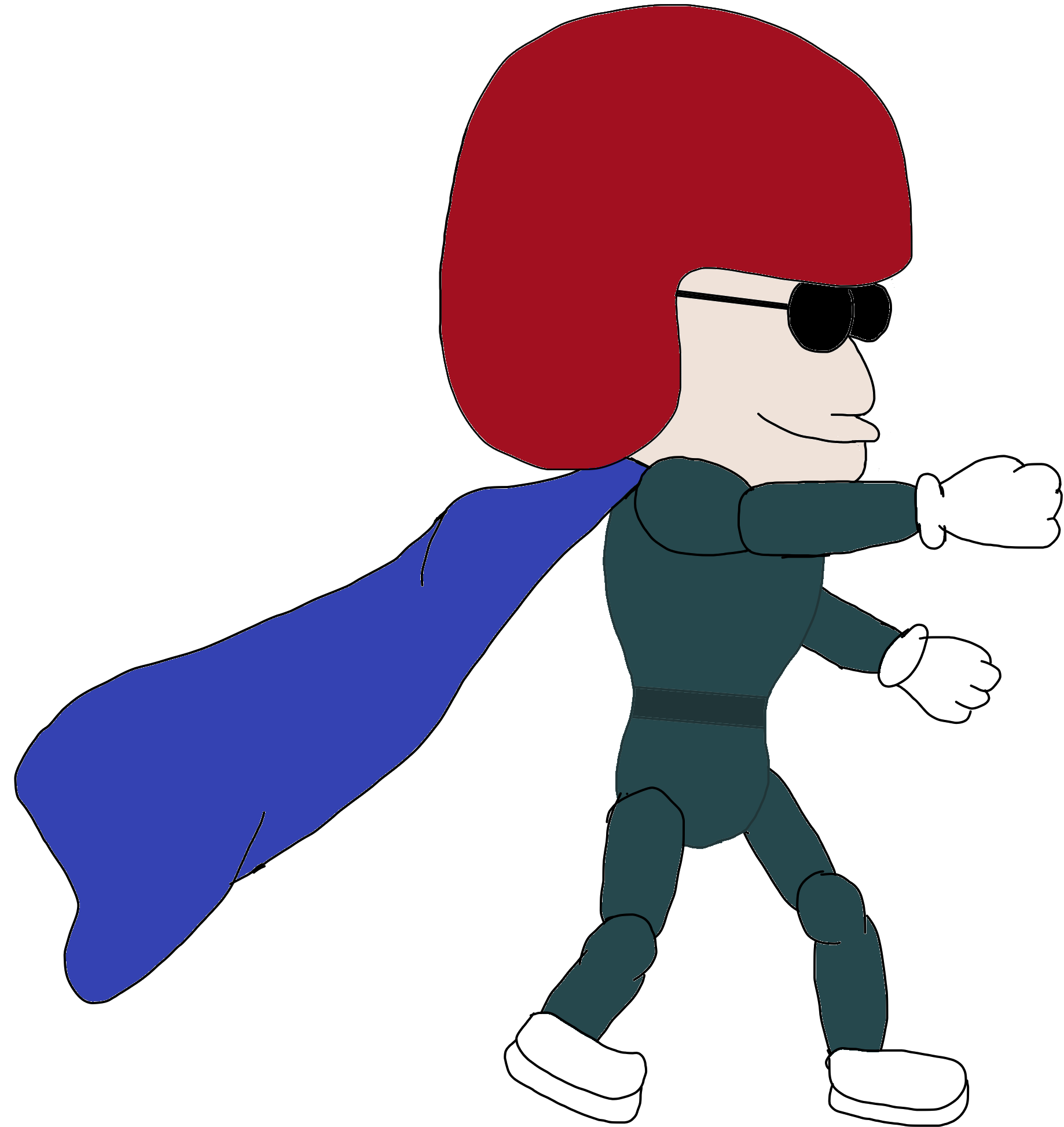
|
About Sean Nelson
I like codes and stuff.
|
1 comments